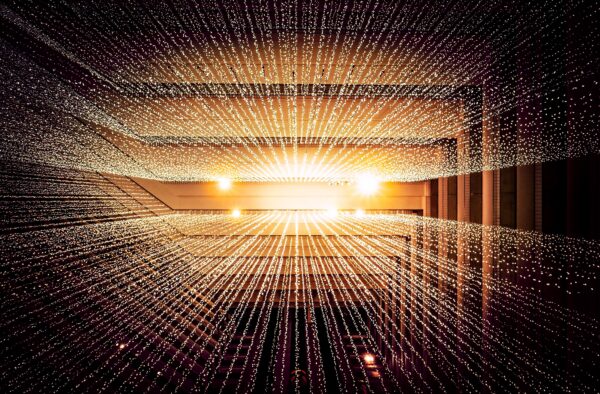
How to pass PHP variables to JavaScript in WordPress
How to pass data from PHP to JS
It’s good practice to put all your data in static strings in your PHP files. If you need to use some data in JavaScript later, it’s also good practice to put your data in your HTML in the form of data-* attributes. But in some cases, you have no choice but to pass strings directly to your JavaScript code. This article will teach you how to properly pass PHP data and static strings to your JavaScript library or file. Consider how to pass data from PHP to JS.
What kind of data might need to be passed data to JS?
Very often, when working with sites built on WordPress, you have to transfer the following data for processing and further work with them in JavaScript files.
- Homepage, admin, plugin, theme or AJAX URLs
- translatable strings (generated dynamically in PHP)
- theme options or WordPress.
The usual way to transfer data.
Let’s say we have a jQuery file called main.js that we want to include in our WordPress site:
var object;
(function($) {
"use strict";
object = {
home: '', // populate this later
pleaseWaitLabel: '', // populate use this later
someFunction: function() {
// code which uses the properties above
}
}
});
We can include it via the functions.php file with the following code:
wp_enqueue_script( 'main', get_template_directory_uri() . '/js/main.js' );
Now the question is how can we populate the home and pleaseWaitLabel properties? You would probably suggest adding the following code to your header.php to get the data you need:
<script>
(function( $ ) {
"use strict";
$(function() {
object.home = '<?php echo get_stylesheet_directory_uri() ?>';
object.pleaseWaitLabel = '<?php _e( 'Please wait..', 'default' ) ?>';
});
}(jQuery));
</script>
And this approach would work great. But there is a more correct approach, which we will now consider.
The old way: using the wp_localize_script function.
The old way to pass data to JavaScript is to use the wp_localize_script function. This function should be used after you have added the script using wp_enqueue_scripts. Let’s look at the syntax of this function and its arguments.
wp_localize_script( $handle, $objectName, $arrayOfValues );
$handle – Refers to the included script to bind values to.
$objectName is a JavaScript object that will contain all the values from $arrayOfValues.
$arrayOfValues - An associative array containing the names and values to be passed to the script.
After calling this function, the $objectName variable becomes available in the specified script. Let’s rewrite the previous example to use a new way of passing data. We first include the script and then we call wp_localize_script in the functions.php file:
wp_enqueue_script( 'main', get_template_directory_uri() . '/js/main.js' );
$dataToBePassed = array(
'home' => get_stylesheet_directory_uri(),
'pleaseWaitLabel' => __( 'Please wait...', 'default' )
);
wp_localize_script( 'main', 'php_vars', $datatoBePassed );
Our home and pleaseWaitLabel values can now be retrieved inside our jQuery file via the php_vars variable. Since we’ve used wp_localize_script, we don’t need to run (or include) anything in our header.php file, and we can safely remove the contents of the <script> tag. We can also remove additional properties from our jQuery script. Now it can be simplified to the following:
var object;
(function($) {
"use strict";
object = {
someFunction: function() {
// code which uses php_vars.home and php_vars.pleaseWaitLabel
}
}
}(jQuery));
New way: using the wp_add_inline_script function
The new and recommended way is to use the wp_add_inline_script function. This function should be used after you have added the script using wp_enqueue_scripts. Let’s look at the syntax and arguments of this function.
wp_add_inline_script( $handle, $data, $position = 'after' );
$handle – The name of the script to which the inline script is added.
$data – A string containing the JavaScript code to be added.
$position – Specifies whether to add the inline script before or after the specified script.
So this function adds an inline script before or after your JavaScript code. Let’s modify the example we covered in the previous paragraph using the wp_add_inline_script version.
wp_enqueue_script( 'main', get_template_directory_uri() . '/js/main.js' );
$dataToBePassed = array(
'home' => get_stylesheet_directory_uri(),
'pleaseWaitLabel' => __( 'Please wait...', 'default' )
);
wp_add_inline_script( 'main', 'const php_vars = ' . json_encode( $dataToBePassed ), 'before' );
Now the home and pleaseWaitLabel values can be accessed inside the jQuery file via the php_vars variable. These variables in JavaScript will be available as php_vars.home and php_vars.pleaseWaitLabel as shown in the following code snippet.
var object;
(function($) {
"use strict";
object = {
someFunction: function() {
console.log(php_vars.home);
console.log(php_vars.pleaseWaitLabel);
}
}
}(jQuery));
Conclusion. How to pass data from PHP to JS
By using wp_add_inline_script , our code has become simpler, and header.php is cleaner, without cumbersome pieces of incomprehensible code. Hope you can use this feature in your code and get the benefits of it. It will also be useful to remember about another method, using the wp_localize_script function, which is also often found in various WordPress themes and plugins.
Leave a Reply