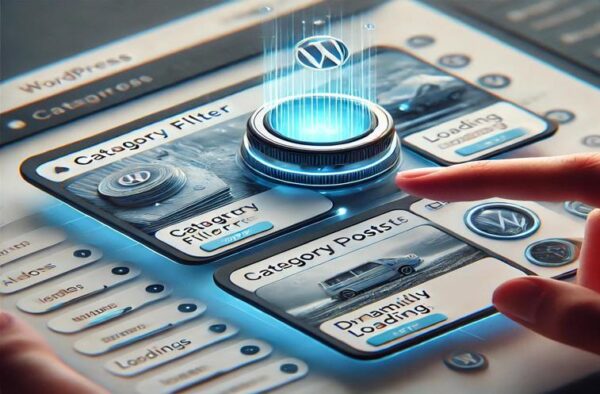
Dynamic AJAX Filtering of Posts in WordPress Without Plugins
Introduction
If your WordPress site contains numerous posts, an efficient content filtering system can greatly enhance the user experience. AJAX filtering allows posts to be dynamically loaded without refreshing the page, significantly improving site speed and usability.
In this article, we will explore how to implement AJAX-based post filtering by category in WordPress without relying on third-party plugins.
Step 1: Creating the Filtering Form in HTML
First, let’s add the HTML code that will generate a dropdown menu with categories.
<div class="ajax-filters">
<form id="ajax-filter">
<?php
$categories = get_terms(array(
'taxonomy' => 'category',
'orderby' => 'name',
));
if ($categories) :
?>
<select id="category-select">
<option value="">Select a category...</option>
<?php foreach ($categories as $category) : ?>
<option value="<?php echo $category->term_id ?>"><?php echo $category->name ?></option>
<?php endforeach; ?>
</select>
<?php endif; ?>
</form>
</div>
<div id="filtered-posts">
<!-- Filtered posts will be loaded here -->
</div>
This code creates a dropdown category filter, allowing users to select a category to filter the posts accordingly.
Step 2: Adding the AJAX Request in JavaScript
Next, we will create a JavaScript function that sends an AJAX request when the category selection changes.
document.addEventListener('DOMContentLoaded', function () {
const categorySelect = document.getElementById('category-select');
const postsContainer = document.getElementById('filtered-posts');
categorySelect.addEventListener('change', function () {
let selectedCategory = categorySelect.value;
let data = new FormData();
data.append('action', 'filter_posts');
data.append('category', selectedCategory);
fetch(ajaxurl, {
method: 'POST',
body: data
})
.then(response => response.text())
.then(data => {
postsContainer.innerHTML = data;
})
.catch(error => console.error('Error:', error));
});
});
This script listens for category selection changes, sends an AJAX request with the selected category, and updates the content dynamically without reloading the page.
Step 3: Processing the AJAX Request in PHP
On the server side, we need to process the AJAX request and return a filtered list of posts based on the selected category.
add_action('wp_ajax_filter_posts', 'ajax_filter_posts');
add_action('wp_ajax_nopriv_filter_posts', 'ajax_filter_posts');
function ajax_filter_posts() {
$category = isset($_POST['category']) ? sanitize_text_field($_POST['category']) : '';
$args = array(
'post_type' => 'post',
'posts_per_page' => -1,
);
if (!empty($category)) {
$args['cat'] = $category;
}
$query = new WP_Query($args);
if ($query->have_posts()) :
while ($query->have_posts()) : $query->the_post();
get_template_part('template-parts/content', get_post_type());
endwhile;
else :
echo '<p>No posts found.</p>';
endif;
wp_die();
}
This PHP code registers an AJAX handler that retrieves and displays posts based on the selected category.
Conclusion
Now, your WordPress site supports AJAX-based post filtering by category without using external plugins. This method greatly enhances content loading speed and user experience.
Key Benefits of This Approach:
✅ Real-time content filtering without reloading the page
✅ Improved site performance and faster response times
✅ Easy integration into any WordPress theme
By following this guide, you can efficiently implement AJAX post filtering, creating a seamless and engaging user experience on your website. 🚀
Leave a Reply